iOS开发之隐形警告(warning),缺陷(bug)整理
iOS invisible warning and bug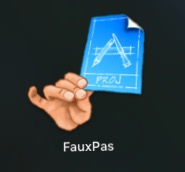
由faus pas检查得来:
1.Weak reference to top-level XIB object
Detail:
IBOutlet references to top-level objects in XIBs should be strong, so as to ensure that the object is not deallocated prematurely.
Read more:
Resource Programming Guide: Managing the Lifetimes of Objects from Nib Files
2.Terminating the app in a release build
Detail:
The exit function is called
Read more:
https://developer.apple.com/library/ios/qa/qa1561/_index.html
3.UI String not localized
Detail:
All strings that are given to APIs that display them in the user interface should be routed through NSLocalizedString() and friends.
self.titleLabel.text = @"预约";
4.XIB refers to unknown resource
Detail:
Resources loaded from disk at runtime should be included in the bundle resources under the correct path. Remember that unlike most Macs (and thus iOS simulators run on those Macs), iOS devices have a case sensitive filesystem.
5.Code refers to unknown resource
Detail:
Resources loaded from disk at runtime should be included in the bundle resources under the correct path. Remember that unlike most Macs (and thus iOS simulators run on those Macs), iOS devices have a case sensitive filesystem.
6.Reserved symbol prefix
Detail:
Two-character prefixes (such as NS) are reserved for Apple’s system frameworks. Your own code should use prefixes that are three characters long.
The CT prefix is used. This prefix is already used in the CoreTelephony / CoreText framework by Apple. The prefixes in your own (or third-party) code should be at least three characters long.
The MC prefix is used. This prefix is already used in the MultipeerConnectivity framework by Apple. The prefixes in your own (or third-party) code should be at least three characters long.
Read more:
https://developer.apple.com/library/ios/documentation/Cocoa/Conceptual/ProgrammingWithObjectiveC/Conventions/Conventions.html
7.Recommended compiler warning options
Detail:
Some compiler warnings and warning groups are so useful that they should be enabled at all times.
8.Assignment of literal zero to pointer
Detail:
Assigning the literal zero to a pointer value might be an indication of a mistake where the programmer actually wanted to assign to an integer-typed member of the pointer type value. Assigning NULL, nil, or Nil instead suppresses warnings from this rule.
Literal zero is assigned to pointer type variable or member. If this is intentional, use NULL, nil, or Nil instead.
9.Retaining or copying delegate
Detail:
To avoid retain cycles, delegate setter methods should not retain or copy the delegate object.
@property (nonatomic, retain) id <VRGCalendarViewDelegate> delegate;
Read more:
https://developer.apple.com/library/ios/documentation/general/conceptual/CocoaEncyclopedia/DelegatesandDataSources/DelegatesandDataSources.html
10.Missing API usage description
Detail:
Some APIs (that access e.g. contacts or calendars) require a usage description in the application metadata. This will be shown to the user when the system asks them to allow the application access to the related user data or system resource. This rule warns when some such APIs are used, but the associated usage description is missing.
The NSPhotoLibraryUsageDescription Info.plist key is strongly recommended but missing.
This key is needed due to the usage of the following code symbols:
ALAssetsLibrary
UIImagePickerControllerSourceTypePhotoLibrary
Read more:
https://developer.apple.com/library/ios/documentation/General/Reference/InfoPlistKeyReference/Articles/CocoaKeys.html#//apple_ref/doc/uid/TP40009251-SW1
11.Literal string for key path
Detail:
Warns when literal strings are used to specify key paths.
Something like NSStringFromSelector(@selector(foo)) is safer because it makes the compiler aware of the selector being specified — this helps find typos at compile time and allows automatic refactoring tools to make appropriate changes.
[manager.requestSerializer willChangeValueForKey:@"timeoutInterval"];
Read more:
nshipster.com/key-value-observing/
12.Complete NSNotificationCenter detachment
Detail:
Warns when an object removes itself as an observer to all notifications (by invoking -[NSNotificationCenter removeObserver:] with self).
This can be a problem if a superclass or a subclass wants to keep observing some notifications. The recommended way to handle this is to remove self as an observer only for the specific notifications that you have previously begun observing.
This rule does not warn about such removals occuring in -dealloc.
[[NSNotificationCenter defaultCenter] removeObserver:self];
Read more:
www.bignerdranch.com/blog/notifications-part-3-gotchas/
13.Conflicting category methods
Detail:
If the name of a method declared in a category is the same as a method in the original class, or a method in another category on the same class (or even a superclass), the behavior is undefined as to which method implementation is used at runtime.
Read more:
https://developer.apple.com/library/ios/documentation/Cocoa/Conceptual/ProgrammingWithObjectiveC/CustomizingExistingClasses/CustomizingExistingClasses.html
14.Assigning delegate property
Detail:
Delegate properties should be declared weak instead of assign. Weak references are safer because they become nil when the referenced object is deallocated.
This rule only applies to code compiled with Automatic Reference Counting (ARC).
@property (assign)id <GetDataDelegate>getDataDelegate;
15.Unused error value
Detail:
Warns when NSError or CFErrorRef variable values are never used.
If you are not interested in the error return value of a method or function, pass NULL for the error argument.
NSError *error;
16.Unnecessary Nib method
Detail:
Warns if -[NSObject awakeFromNib] is implemented in a class that is not archived in any known XIB.
17.Undetached delegate or data source
Detail:
When an object sets itself as the delegate or data source of one of its members, it must detach that reference in its -[NSObject dealloc] method.
18.Globally caching a thread-unsafe class instance
Detail:
Warns about variables with global storage whose type is an object pointer to a known thread-unsafe class.
-[NSThread threadDictionary] can be used to cache instances of thread-unsafe classes — one instance for each thread.
This rule does not warn about code occuring in subclasses of (or categories on) UIKit or AppKit classes, because it can be assumed that these are always accessed solely from the main thread.
Read more:
https://developer.apple.com/library/ios/documentation/Cocoa/Conceptual/Multithreading/ThreadSafetySummary/ThreadSafetySummary.html
19.Fragile error condition check
Detail:
Methods that pass back NSError objects by reference return NO or nil to indicate an error condition. This rule warns if the NSError pointer is checked against nil without checking the return value.
Read more:
https://developer.apple.com/library/ios/DOCUMENTATION/Cocoa/Conceptual/ErrorHandlingCocoa/CreateCustomizeNSError/CreateCustomizeNSError.html#//apple_ref/doc/uid/TP40001806-CH204-SW1
https://developer.apple.com/library/ios/documentation/Cocoa/Conceptual/ProgrammingWithObjectiveC/ErrorHandling/ErrorHandling.html#//apple_ref/doc/uid/TP40011210-CH9-SW5
https://github.com/NYTimes/objective-c-style-guide#error-handling
20.Unprefixed category method
Detail:
Category methods on system classes must be prefixed in order to avoid name collisions.
By default, this rule considers a method name prefixed if it begins with at least three lowercase characters (or the lowercase version of the ‘Class Prefix’ configured for the project), followed by an underscore.
Read more:
https://developer.apple.com/library/ios/documentation/Cocoa/Conceptual/ProgrammingWithObjectiveC/CustomizingExistingClasses/CustomizingExistingClasses.html
21.Missing NSNotificationCenter observer detachment
Detail:
You must invoke -[NSNotificationCenter removeObserver:] or -[NSNotificationCenter removeObserver:name:object:] before the observer object is deallocated.
This rule only considers cases where the observer reference is self, or an instance variable or an Objective-C property in self.
This only applies to iOS 8 (and earlier) and OS X 10.10 (and earlier).
22.Fixed-format NSDateFormatter not using invariant (POSIX) locale
Detail:
Warns when an NSDateFormatter is used with fixed-format dates without using the invariant en_US_POSIX locale. If any other locale is used, the date format string may be overwritten, depending on system date and time settings.
When working with user-visible dates, date and time styles should be used instead of setting a date format.
Read more:
https://developer.apple.com/library/ios/qa/qa1480/_index.html
https://developer.apple.com/library/mac/documentation/Cocoa/Conceptual/DataFormatting/Articles/dfDateFormatting10_4.html#//apple_ref/doc/uid/TP40002369-SW7
23.Unidiomatic accessor naming
Detail:
Warns if getter methods are named in the form getSomething instead of something.
@property (assign)id <GetDataDelegate>getDataDelegate;
24.Reserved symbol prefix
Detail:
Two-character prefixes (such as NS) are reserved for Apple’s system frameworks. Your own code should use prefixes that are three characters long.
The JK prefix is used. Two-character prefixes are reserved for Apple’s system frameworks. The prefixes in your own (or third-party) code should be at least three characters long.
Read more:
https://developer.apple.com/library/ios/documentation/Cocoa/Conceptual/ProgrammingWithObjectiveC/Conventions/Conventions.html
25.Duplicate resource
Detail:
Warns if two resource files are equal.
26.Recommended project settings
Detail:The ‘Organization’ and ‘Class Prefix’ settings should always be set. The expected values for other settings are configurable.
27.Using -[UIViewController initWithNibName:bundle:] outside the UIViewController implementation
Detail:
It is not advisable to use -[UIViewController initWithNibName:bundle:] to instantiate UIViewController subclasses outside of the subclass implementation itself. This breaks encapsulation (the subclass should be the one to decide which NIB to use) and makes it more likely that a typo in the NIB name string will crash the app during runtime.
28.Unprefixed Objective-C class
Detail:
Warns when an Objective-C class name has no prefix (e.g. Thing instead of FPXThing).
29.Uncommented localized string
Detail:
Warns if NSLocalizedString() or one of its variants is used without a non-empty comment argument.
30.Setter invocation in init or dealloc method
Detail:
It is recommended to avoid invoking setter methods on self in init methods, or in -[NSObject dealloc]. Doing so might trigger KVC notifications, which others might observe, and expect the object to be in a valid, consistent state.
Read more:
https://developer.apple.com/library/ios/documentation/cocoa/conceptual/ProgrammingWithObjectiveC/EncapsulatingData/EncapsulatingData.html#//apple_ref/doc/uid/TP40011210-CH5-SW11
stackoverflow.com/questions/5094615/calling-a-method-on-self-while-in-dealloc
31.Unexpected retina image resolution
Detail:
The retina (‘@2x’ or ‘@3x’) version of an image resource must be exactly double or triple the size of the corresponding low-resolution image (assuming the point size of the presentation context remains the same regardless of screen resolution). Otherwise the effective resolution of the image will not match the effective resolution of its presentation context, which will lead to a blurry image, empty space at the edges, or overflow.
Read more:
https://developer.apple.com/high-resolution/
31.Non-copying property of immutable NSCopying type
Detail:
Warns if “retaining” semantics are specified in a @property declaration for a common immutable NSCopying class type that also has a mutable subclass variant (for example NSString).
This rule helps avoid situations where the value of a property could change without the setter being invoked (i.e. the object gets mutated).
The progrees property is declared with strong semantics. This should be copy instead.
@property (nonatomic , strong) NSString *progrees;
31.Old, verbose Objective-C syntax
Detail:
Warns if Objective-C literals, boxed expressions and subscript notation are not used whenever possible.
Note that Xcode can automatically fix your code to do this (see the Edit → Refactor → Convert To Modern Objective-C Syntax… menu item).
Read more:
clang.llvm.org/docs/ObjectiveCLiterals.html
32.Null coalescing operator usage
Detail:
Expressions of the form (obj ? obj : other) should be written as obj ?: other.
Please note that in the form (obj ? obj : other) the obj expression is evaluated twice, while in the obj ?: other form it is evaluated only once.
33.Missing image resolution variant
Detail:
Warns when either the high-resolution (@2x or @3x) or the low-resolution variant of an image resource is missing.
Configure this rule
34.Hardcoded self class reference
Detail:
Warns if an Objective-C class (or an instance of a class) sends a message to its own class object using a hardcoded reference to the class, e.g. [FOOThing alloc].
It is recommended to use self instead (e.g. [self alloc] or [[self class] alloc]) so that the concrete class would receive the message, and subclassing behavior would not be impeded.
Read more:
qualitycoding.org/factory-method/
https://developer.apple.com/library/ios/releasenotes/ObjectiveC/ModernizationObjC/AdoptingModernObjective-C/AdoptingModernObjective-C.html#//apple_ref/doc/uid/TP40014150-CH1-SW2
35.Modifying the value of an argument variable
Detail:
Argument variable values should not be modified directly — code is easier to follow if you can always trust that argument variable values do not change.
36.Macro-based include guard
Detail:
Simplify the header by replacing the macro definition check -based include guard with the ‘once’ pragma.
Read more:
en.wikipedia.org/wiki/Pragma_once
37.Constructor return type
Detail:
Warns if an init or factory method return type is anything other than instancetype.
Properly specifying the return type for factory methods (e.g. +[NSArray arrayWithObject:]) allows the compiler to perform more type checking, which helps catch bugs. Even though the compiler automatically determines the return types of init methods (even if they are declared as id) it is still good to be explicit and use instancetype.
Read more:
http://stackoverflow.com/questions/8972221/would-it-be-beneficial-to-begin-using-instancetype-instead-of-id
转载请注明:天狐博客 » iOS开发之隐形警告(warning)与缺陷(bug)大全